Writing a tile server in Python
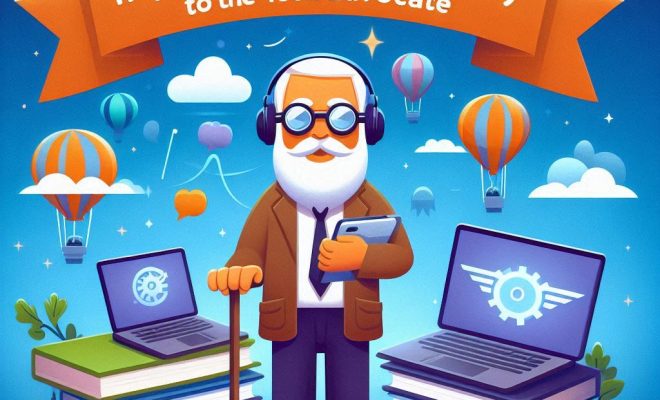
In the world of web mapping, tile servers are the silent heroes. They break down massive geospatial datasets into bite-sized, readily accessible tiles, making map rendering fast and efficient. This article explores the fascinating world of building your own tile server in Python, empowering you to create and customize your own map experiences.
Why Build a Tile Server?
Control and Customization: You’re in charge of the entire process, from data source to map style, giving you unparalleled control over the final product.
Performance Optimization: Tile servers pre-render data, ensuring rapid map loading and seamless user interaction, even with complex datasets.
Data Flexibility: You can use diverse data sources, from open-source geospatial datasets to your own custom data, bringing unique perspectives to your maps.
Learning Opportunity: Building your own tile server provides valuable insight into the core principles of web mapping and data processing.
The Python Ecosystem for Tile Servers
Python boasts a robust ecosystem specifically designed for building tile servers. Key players include:
1. GeoDjango: A powerful framework for building geospatially-enabled web applications.
2. TileStache: A flexible and highly customizable tile server framework.
3. Mapnik: A renowned library for rendering high-quality vector maps.
4. GDAL/OGR: A library for reading and writing geospatial data formats.
A Step-by-Step Guide
Choose your framework: Start with a framework like TileStache for its simplicity and flexibility.
Prepare your data: Use GDAL/OGR to convert your geospatial data into a format compatible with your chosen framework (e.g., GeoTIFF, Shapefile).
Define map styles: Use a styling language like Mapnik’s XML-based syntax to define how your map elements (points, lines, polygons) are visualized.
Configure your tile server: Set up your tile server using your chosen framework, specifying data sources, styles, and rendering parameters.
Serve tiles: Run your tile server and access the generated tiles through a web browser or map application.
Example TileStache Setup
“`python
from TileStache import
config = {
‘cache’: {
‘name’: ‘disk’,
‘path’: ‘/path/to/cache’
},
‘layers’: {
‘my_layer’: {
‘provider’: {
‘name’: ‘mapnik’,
‘mapfile’: ‘/path/to/mapfile.xml’
},
‘metatile’: 4,
‘extension’: ‘png’,
‘mime_type’: ‘image/png’
}
}
}
app = App(config)
“`
This simple example demonstrates the core components of a TileStache setup, showcasing the use of a Mapnik provider for rendering and a disk-based cache for efficient tile storage.
Embark on Your Tile Server Journey
Building your own tile server offers a rewarding journey, enabling you to craft custom, high-performance mapping experiences. By leveraging the powerful capabilities of Python and its geospatial libraries, you can unlock the potential of your data and create compelling visualizations for diverse applications. So, dive in, explore the world of tile servers, and let your creativity flow through the pixels of your own map creations.