ps aux written in bash without forking
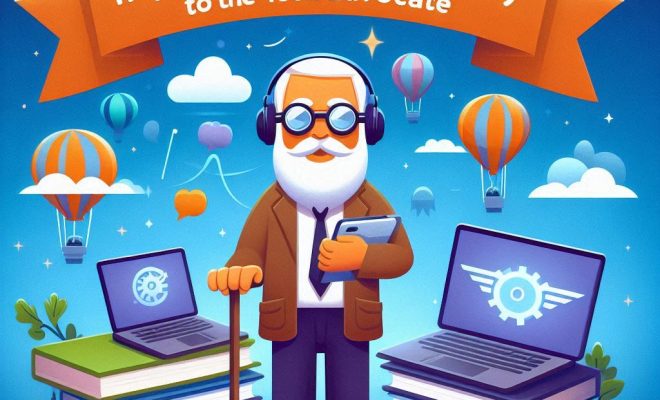
ps aux` is one of the most commonly used commands in Unix-like operating systems for monitoring active processes. It provides a snapshot of current processes along with useful information such as Process ID (PID), user, CPU usage, memory usage, and more. But what if we wanted to replicate the functionality of `ps aux` in the Bash shell without forking new processes? This article will guide you through understanding processes, the purpose of `ps aux`, and how to achieve similar results using built-in Bash features.
Understanding Processes
Before we dive into the mechanics of `ps aux`, it’s crucial to understand what a process is. A process is an instance of a running program. Each process in the system is assigned a unique PID, and it can be executed in the foreground or background. The operating system provides various system calls to interact with these processes.
The `ps` command is used to report a snapshot of current processes. The `a`, `u`, and `x` options together represent the following:
– a: Show processes for all users.
– u: Display user-oriented format.
– x: Show processes not attached to a terminal.
When combined, `ps aux` provides a comprehensive listing of all running processes with detailed information.
Limitations of Forking
Forking is the process of creating a new process by duplicating the existing one. Although this is the common method utilized in Unix-like systems for executing a new command, it may not always be desired. For instance, forking can be resource-intensive and can lead to overhead if frequently invoked in scripts or tools where efficiency is critical.
Mimicking `ps aux` in Bash without Forking
While replicating the full functionality of `ps aux` without using any forks is quite challenging, we can utilize built-in Bash features, such as reading from `/proc`, to retrieve similar information. The `/proc` filesystem contains a wealth of details about running processes, organized by their corresponding PIDs.
Reading from `/proc`
The `/proc` filesystem provides a virtual representation of process information. Each process running on the system has its directory within `/proc`, named by its PID. For example, the following information is available in each PID’s directory:
– `cmdline`: The command line that started the process.
– `stat`: Provides status information about the process.
– `status`: Offers detailed information about the process, including user, memory usage, and more.
Sample Bash Script
To retrieve information similar to that from `ps aux`, we can build a simple Bash script that reads the information from `/proc`. Here’s an example of how you can achieve this:
“`bash
!/bin/bash
Print the header similar to ‘ps aux’
printf “%-10s %-10s %-10s %-10s %s\n” USER PID %CPU %MEM CMD
Iterate through process directories in /proc
for pid in /proc/[0-9]; do
if [[ -d $pid ]]; then
Extract PID
pid_number=”${pid/}”
Read user information
user=”$(ps -o user= -p $pid_number)”
Read CPU and memory usage
cpu_mem_info=”$(awk ‘{printf “%s %s”, $3, $4}’ $pid/stat)”
Read command line
cmd=”$(tr ‘\0’ ‘ ‘ < $pid/cmdline)”
Print the information
printf “%-10s %-10s %-10s %-10s %s\n” “$user” “$pid_number” $cpu_mem_info “$cmd”
fi
done
“`
Explanation of the Script
– The script first prints a header similar to `ps aux`.
– It iterates over directories in `/proc` that match the pattern of numeric PID directories.
– For each PID:
– It retrieves the username using `ps -o user=` to avoid forking a new shell too often.
– It extracts CPU and memory usage from the `stat` file.
– It retrieves the command line used to start the process from the `cmdline` file while translating null characters to spaces.
– Finally, it prints the gathered information in a formatted manner.
Limitations
While this script offers a functional equivalent to `ps aux`, it contains limitations. It is less efficient and less feature-complete and might require finer tuning to handle edge cases. Moreover, it may not match the very latest features available in `ps` commands, such as filtering or formatting options.
Conclusion
Using `/proc`, it is possible to retrieve process information without forking new processes in Bash, though this method can be less elegant and efficient. By understanding how the system maintains process information and utilizing built-in file reading methods, we can still monitor system activities effectively. While tools like `ps aux` are highly optimized and versatile, this exercise highlights how you can work within the constraints of a built-in programming environment like Bash.