Postgres Feature You’re Not Using – Ctes A.K.A. WITH Clauses
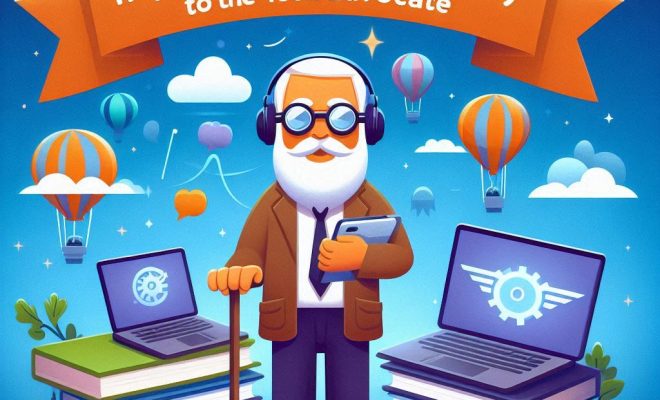
Have you ever felt like your SQL queries were turning into spaghetti code? I certainly have! But fear not, fellow data wranglers, because today we’re diving into one of PostgreSQL‘s most powerful yet underutilized features: Common Table Expressions (CTEs), also known as WITH clauses. Trust me, this is a game-changer you won’t want to miss!
What Are CTEs, and Why Should You Care?
CTEs are like your query’s secret weapon. They allow you to define named subqueries that you can reference multiple times within your main query. Think of them as temporary views that exist only for the duration of your query. Here’s the basic syntax:
WITH cte_name AS (
— Your subquery here
)
SELECT * FROM cte_name;
But why should you care? Well, let me tell you about the time CTEs saved my bacon on a complex data analysis project…
Real-World CTE Magic: A Personal Anecdote
Picture this: I was knee-deep in a project analyzing customer behavior across multiple touchpoints. The queries were getting more complex by the minute, and my code was starting to look like a plate of overcooked noodles. That’s when CTEs came to my rescue!
Let’s look at a simplified example:
WITH customer_touchpoints AS (
SELECT customer_id,
COUNT(DISTINCT channel) AS channel_count,
MAX(interaction_date) AS last_interaction
FROM interactions
GROUP BY customer_id
),
high_value_customers AS (
SELECT customer_id
FROM orders
GROUP BY customer_id
HAVING SUM(order_value) > 10000
)
SELECT ct.*,
CASE WHEN hvc.customer_id IS NOT NULL THEN ‘High Value’ ELSE ‘Regular’ END AS customer_type
FROM customer_touchpoints ct
LEFT JOIN high_value_customers hvc ON ct.customer_id = hvc.customer_id;
This query uses two CTEs to break down complex logic into manageable, reusable pieces. It’s like Marie Kondo for your SQL – sparking joy with every clean, organized line!
The Benefits of Embracing CTEs
Readability: CTEs make your queries self-documenting. Each CTE can be given a descriptive name, making the overall query easier to understand.
Maintainability: Need to modify a subquery used in multiple places? With CTEs, you only need to change it in one place!
Performance: In some cases, CTEs can improve query performance by allowing the database to optimize complex queries more effectively.
Recursion: CTEs support recursive queries, opening up a whole new world of possibilities for hierarchical or graph-like data structures.
Common Pitfalls and How to Avoid Them
While CTEs are powerful, they’re not a silver bullet. Here are a couple of things to watch out for:
Overuse: Don’t go CTE-crazy! Use them when they genuinely improve readability or are necessary for recursion.
Performance assumptions: CTEs are optimized differently in different database systems. In Postgres, they’re generally materialized, which can impact performance for very large datasets.