Lesser known parts of Python standard library – Trickster Dev
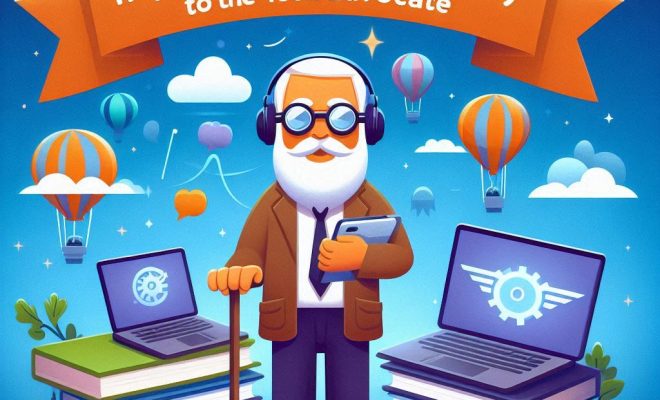
1. timeit: Measuring code execution time is crucial for optimization. `timeit` allows you to time code snippets, compare different approaches, and identify performance bottlenecks.
2. collections.namedtuple: Tired of working with tuples and remembering their indices? namedtuple lets you create tuples with named fields, improving readability and maintainability.
3. functools.partial: Need to create specialized versions of functions with pre-filled arguments? `partial` allows you to partially apply arguments, making your code cleaner and more reusable.
4. contextlib.contextmanager: For managing resources like files or network connections, `contextmanager` provides a concise way to ensure proper setup and cleanup, even in case of exceptions.
5. itertools: This module offers a wealth of tools for working with iterators, including functions for generating sequences, filtering, and combining iterables.
6. asyncio: For asynchronous programming, `asyncio` lets you handle multiple tasks concurrently without blocking the main thread, making your applications more responsive and efficient.
7. statistics: Beyond basic calculations, `statistics` offers a wide range of statistical functions for calculating mean, median, variance, and more.
8. pprint: When dealing with complex data structures, `pprint` helps you prettily print them for easier readability and debugging.
9. argparse: Crafting user-friendly command-line interfaces is a breeze with `argparse`. It simplifies argument parsing and validation, allowing users to interact with your scripts seamlessly.
10. logging: Logging is crucial for debugging and monitoring your applications. `logging` provides a robust framework for capturing and recording events at different severity levels.
These are just a few examples of the many hidden treasures in the Python standard library. Explore them and discover how they can empower you to write more efficient, readable, and maintainable code.