Introduction to WebAssembly in Go
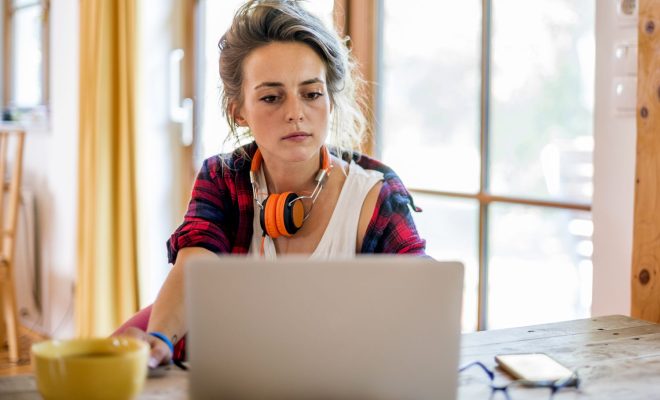
WebAssembly (often abbreviated as WASM) is a low-level binary format used for deploying code on the web. This new web technology has become increasingly popular because it allows developers to write code in languages other than JavaScript that can be executed in the browser. In this article, we will explore how to use WebAssembly in Go and introduce some of the benefits it offers.
What is WebAssembly?
WebAssembly is a binary format that can be compiled from programming languages such as C, C++, Rust, and others. It is designed to be lightweight and efficient and to run in a secure sandbox environment. This means that, unlike traditional JavaScript programs, WebAssembly code is run directly by the browser’s engine, dramatically improving performance.
WebAssembly was first introduced in 2017 as a standard by the World Wide Web Consortium (W3C). Since then, it has gained popularity among developers because it allows them to reuse their existing codebase written in other programming languages.
Why use WebAssembly in Go?
Go is a popular programming language that is widely used for building high-performance backend web applications. However, the language is not as well suited for developing frontend applications. This is because JavaScript is currently the dominant language for building frontend web applications, and it is not easy to integrate Go code with JavaScript.
The use of WebAssembly in Go helps to solve this problem. By using WebAssembly, Go developers can now write frontend applications in Go, which can be executed in the browser alongside JavaScript. This enables developers to leverage Go’s powerful backend capabilities to build full-stack web applications.
How to use WebAssembly in Go?
To use WebAssembly in Go, you need to install two tools: a WebAssembly compiler and a utilities package. The most popular WebAssembly compiler for Go is TinyGo, which can be installed using the following command:
“`
$ go get -u tinygo.org
“`
The utilities package can be installed using the following command:
“`
$ go get -u github.com/WebAssembly/wasi-sdk
“`
Once you have installed these tools, you can start writing your WebAssembly code in Go. Here is a simple example of how to use WebAssembly in Go:
“`
package main
import (
“fmt”
“syscall/js”
)
func main() {
c := make(chan bool)
js.Global().Set(“add”, js.FuncOf(add))
<-c
}
func add(this js.Value, args []js.Value) interface{} {
num1 := args[0].Int()
num2 := args[1].Int()
return num1 + num2
}
“`
In this example, we are defining a function that adds two numbers and returning the result as an interface. We then set the function as a global variable in the JavaScript environment using the `js.Global().Set()` function.
Conclusion
WebAssembly is a powerful technology that enables developers to write frontend web applications in languages other than JavaScript. By using WebAssembly in Go, you can leverage Go’s powerful backend capabilities to build full-stack web applications. With the increasing popularity of WebAssembly, we expect to see more Go developers adopting this new technology in the coming years.