IF Statements to Use for Smarter Windows Batch Scripts
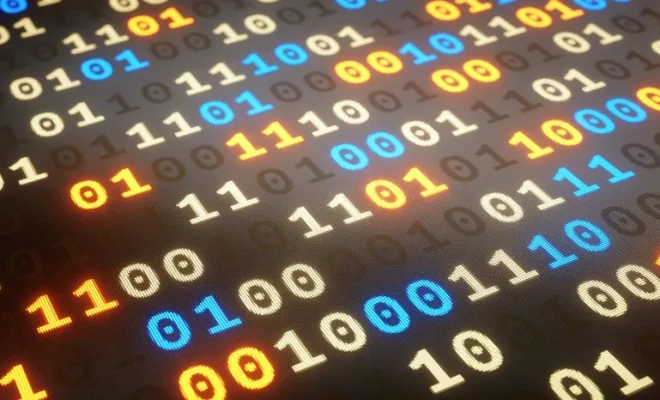
Windows Batch Scripts are an incredibly useful tool for automating tasks and saving time, but they can quickly become complicated and difficult to manage. One way to simplify and streamline your batch scripts is by using IF statements. In this article, we will explore the different types of IF statements that you can use to create smarter Windows Batch Scripts.
IF Statements in Basics:
IF statements are conditional statements that allow you to execute certain commands only if a specific condition is met. The syntax for a basic IF statement is as follows:
“`
IF condition command
“`
Where ‘condition’ is the condition that must be met, and ‘command’ is the command that will be executed if the condition is true.
For example, if you only want a command to be executed if a certain file exists, you can use the following IF statement:
“`
IF EXIST “C:\example\file.txt” command
“`
This will only execute the ‘command’ if the file ‘file.txt’ exists in the directory ‘C:\example’.
Conditional Operators:
IF statements can become much more complex by combining multiple conditions using conditional operators. The most common conditional operators are:
– ‘==’ – equals
– ‘!==’ – not equals
– ‘<’ – less than
– ‘>’ – greater than
– ‘<=’ – less than or equal to
– ‘>=’ – greater than or equal to
For example, if you want to execute a command only if a variable called ‘x’ is greater than 10, you can use the following IF statement:
“`
IF %x% GTR 10 command
“`
This will only execute the ‘command’ if the value of ‘x’ is greater than 10.
Using ELSE in IF Statements:
Another useful feature of IF statements is the ELSE statement. The ELSE statement allows you to specify a command that will be executed if the condition in the IF statement is false. The basic syntax for an IF/ELSE statement is as follows:
“`
IF condition (
command1
) ELSE (
command2
)
“`
For example, if you want to execute one command if ‘x’ is greater than 10, and a different command if it is not, you can use the following IF/ELSE statement:
“`
IF %x% GTR 10 (
command1
) ELSE (
command2
)
“`
This will execute ‘command1’ if the value of ‘x’ is greater than 10, and ‘command2’ if the value of ‘x’ is less than or equal to 10.
Using IF Statements in Loop:
IF statements can also be used within loops to create smarter batch scripts. Using IF statements in a loop can help you check for specific conditions and execute commands based on those conditions.
For example, if you want to loop through all the files in a directory and execute a command only if a certain file exists, you can use the following FOR loop with an IF statement:
“`
FOR %%f IN (*.txt) DO (
IF EXIST “C:\example\%%f” (
command
)
)
“`
This will loop through all the files in the directory with the ‘.txt’ extension, and execute the ‘command’ only if the file exists in the ‘C:\example’ directory.
Conclusion:
In conclusion, IF statements are a powerful tool that can be used to create smarter Windows Batch Scripts. They allow you to execute commands only if specific conditions are met, making your scripts more efficient and easier to manage. By combining multiple conditions using conditional operators and using IF statements in loops, you can create more complex and dynamic batch scripts that can save you time and energy.