How to Split a String in Python
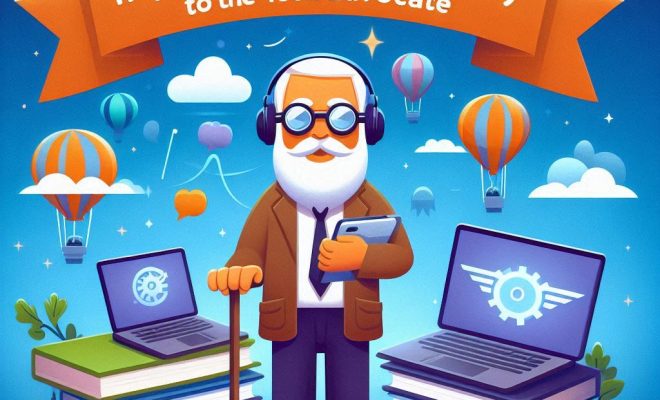
Python is a popular programming language that supports a range of string manipulation functions. By splitting a string in Python, you can break down a string into smaller parts based on a specified delimiter. In this article, we will explore how to split a string in Python.
Method 1: Using the split() function
The split() function in Python can be used to split a string into multiple parts based on a specified delimiter. The syntax of the split() function is as follows:
string.split(delimiter, maxsplit)
The first parameter, delimiter, specifies the character or substring where the string should be split. The second parameter, maxsplit, specifies the maximum number of splits that can be made. If this parameter is not specified, all occurrences of the delimiter will be used to split the string.
Here is an example of how to use the split() function to split a string in Python:
string = “apple,banana,cherry”
fruits = string.split(“,”)
print(fruits)
Output:
[‘apple’, ‘banana’, ‘cherry’]
In this example, the split() function is used to split the string “apple,banana,cherry” using a comma (“,”) as the delimiter. The function returns a list of three strings: “apple”, “banana”, and “cherry”.
Method 2: Using the rsplit() function
The rsplit() function in Python allows you to split a string from the right side. The syntax of the rsplit() function is the same as the split() function, except that it splits the string from the right side.
Here is an example of how to use the rsplit() function in Python:
string = “apple,banana,cherry”
fruits = string.rsplit(“,”, 1)
print(fruits)
Output:
[‘apple,banana’, ‘cherry’]
In this example, the rsplit() function is used to split the string “apple,banana,cherry” using a comma (“,”) as the delimiter, starting from the right side of the string. The 1 in the second parameter specifies that the string should be split into two parts.
Method 3: Using regular expressions
Python also supports regular expressions for string manipulation. The re module in Python provides functions to split a string based on a regular expression pattern.
Here is an example of how to use the re module to split a string in Python:
import re
string = “apple,banana,cherry”
fruits = re.split(“,”, string)
print(fruits)
Output:
[‘apple’, ‘banana’, ‘cherry’]
In this example, the re.split() function is used to split the string “apple,banana,cherry” using a comma (“,”) as the delimiter. The function returns a list of three strings: “apple”, “banana”, and “cherry”.