How to Send Emails From an Excel Spreadsheet Using VBA Scripts
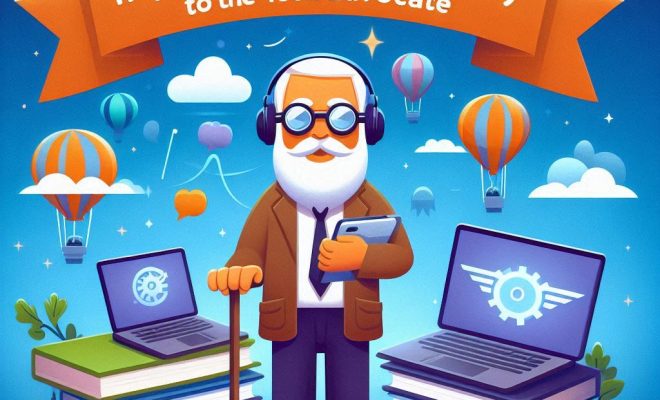
Excel is a powerful tool for organizing and analyzing data. But did you know that you can also use it to send emails? By writing a VBA script, you can automate the process of sending emails from your Excel spreadsheet. Here’s how to do it.
Step 1: Create a new module in VBA
First, you need to create a new module in your Excel workbook. To do this, open the Visual Basic Editor by pressing Alt+F11. Then, click on Insert and select Module. Name the module whatever you like.
Step 2: Define the email properties
Next, you need to define the properties of the email you want to send. This includes the recipient, subject, body, and any attachments you want to include.
You can do this by creating variables for each property. Here’s an example:
Dim EmailTo As String
Dim EmailSubject As String
Dim EmailBody As String
Dim EmailAttachment As String
EmailTo = “[email protected]”
EmailSubject = “Test email”
EmailBody = “This is a test email.”
EmailAttachment = “C:\Path\To\Attachment.pdf”
Step 3: Create the email object
Once you’ve defined the email properties, you need to create an email object using the CreateObject() method. Here’s how you can do it:
Dim OutApp As Object
Dim OutMail As Object
Set OutApp = CreateObject(“Outlook.Application”)
Set OutMail = OutApp.CreateItem(0)
This creates a new instance of Microsoft Outlook and sets the type of item to be created to a new email message.
Step 4: Set the email properties
Now that you have the email object, you need to set its properties based on the values you defined earlier. Here’s how to do it:
With OutMail
.To = EmailTo
.Subject = EmailSubject
.HTMLBody = EmailBody
.Attachments.Add EmailAttachment
.Send
End With
This sets the recipient, subject, body, and attachment fields of the email object. The .Send method sends the email immediately.
Step 5: Test the script
Finally, you can test the script by running it within the Visual Basic Editor. Click on the Run button (or press F5) to execute the script.
If everything is set up correctly, you should see a new email window open up in Outlook with the properties you defined earlier. Verify that the email looks the way you want it to, and then click on the Send button to send it.