How to Save Data to a CSV File in a C# Application
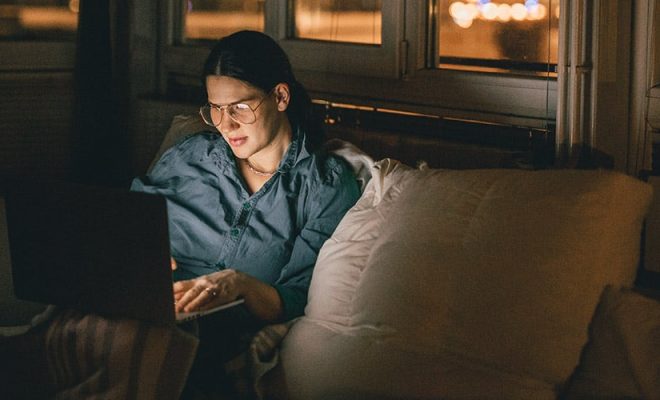
Saving data in a CSV file is a simple and popular way to store and share data. A CSV file, also known as a Comma Separated Value file, is a plain text file that uses commas to separate values. In this article, we will discuss how to save data to a CSV file in a C# application.
Step 1: Create a new CSV file
First, we need to create a new CSV file to save our data. We can create a CSV file using any text editor or spreadsheet software. Alternatively, we can create a new CSV file programmatically using C#.
To create a new CSV file programmatically, we can use the StreamWriter class, which is included in the System.IO namespace. We can create a new StreamWriter object and pass the file path and name as a parameter. Here is an example:
“`
StreamWriter writer = new StreamWriter(“data.csv”);
“`
Step 2: Write data to the CSV file
After creating a new CSV file, we can write data to the file using the StreamWriter object. We can use the WriteLine method to write a line of text to the file. To separate the values in each line, we can use commas. Here is an example:
“`
writer.WriteLine(“Name, Age, Gender”);
writer.WriteLine(“John, 25, Male”);
writer.WriteLine(“Jane, 30, Female”);
“`
In this example, we first write a line that contains the column headers. Then, we write two lines of data, each containing a name, age, and gender separated by commas.
Step 3: Close the CSV file
After writing the data, we need to close the CSV file. We can use the Close method of the StreamWriter object to do this. Here is an example:
“`
writer.Close();
“`
This will ensure that any data still in the buffer is written to the CSV file before it is closed.
Final thoughts
Saving data to a CSV file in a C# application is a simple process. By creating a new CSV file, writing data to it, and closing the file, we can save data in a format that is easy to share and manipulate. The StreamWriter class is a powerful tool that allows us to programmatically create and write to CSV files in our C# applications.