How to Memoize Functions and Values in JavaScript and React
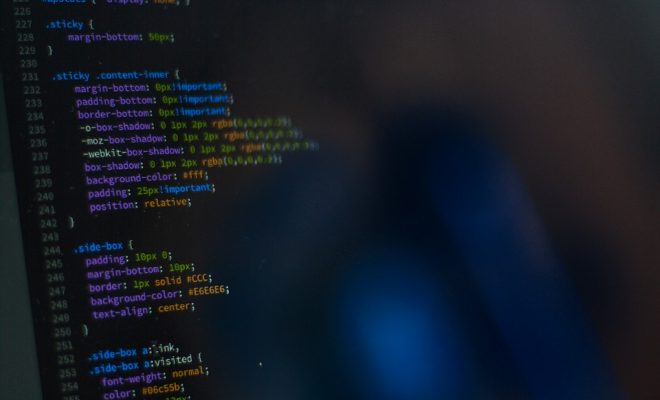
Memoization is a technique that can significantly improve performance by caching expensive operations and returning the cached result instead of recomputing it. In JavaScript and React, memoization can be used on both functions and values to optimize the rendering process and reduce unnecessary calculations.
In this article, we will explore how to memoize functions and values in JavaScript and React and look at some use cases where memoization can be particularly helpful.
Memoizing Functions
When we memoize a function, we create a cached version of the function that returns the cached result instead of recomputing the function’s output every time it is called. In JavaScript, this can be achieved using closures and the concept of cache.
Here’s an example of how we can memoize a function in JavaScript:
“`
function memoize(func) {
let cache = {};
return function(…args) {
let argString = JSON.stringify(args);
if (cache[argString]) {
return cache[argString];
} else {
let result = func.apply(this, args);
cache[argString] = result;
return result;
}
};
}
function add(a, b) {
return a + b;
}
const memoizedAdd = memoize(add);
console.log(memoizedAdd(2, 3)); // Output: 5
console.log(memoizedAdd(2, 3)); // Output: 5 (cached)
“`
In this example, the `memoize` function takes a function as an argument and returns a new function that memoizes the original function by creating a cache object to store the arguments and results. If the function is called again with the same arguments, the cached result is returned instead of recomputing the function’s output.
Memoizing Values
Memoizing values can also be useful in situations where the same value is used multiple times, and you want to avoid recomputing it. In React, for example, memoized values can be used to avoid unnecessary re-renders of components.
Here’s an example of how we can memoize a value in React:
“`
import React, { useMemo } from “react”;
function MyComponent({ value1, value2 }) {
const memoizedValue = useMemo(() => {
// Expensive computation
return value1 + value2;
}, [value1, value2]);
return
{memoizedValue}
;
}
“`
In this example, we use the React `useMemo` hook to memoize the expensive computation that calculates `value1` and `value2`. By passing the dependencies `[value1, value2]`, we ensure that the memoized value is only recomputed when either `value1` or `value2` changes.
Use Cases for Memoization
Memoization can be particularly helpful in scenarios where expensive operations are repeated frequently, such as making API requests, performing complex calculations, or rendering large lists.
In addition to optimizing performance, memoization can also simplify code and reduce complexity by separating expensive computations from the main application logic.
Conclusion
Memoization is a powerful technique that can help optimize performance in JavaScript and React applications by caching expensive operations and returning the cached result instead of recomputing it. Whether we’re memoizing functions or values, the goal is to improve the efficiency of our code and reduce unnecessary computations. By leveraging memoization, we can make our applications run faster and smoother, resulting in a better user experience for our users.