How to make a calculator
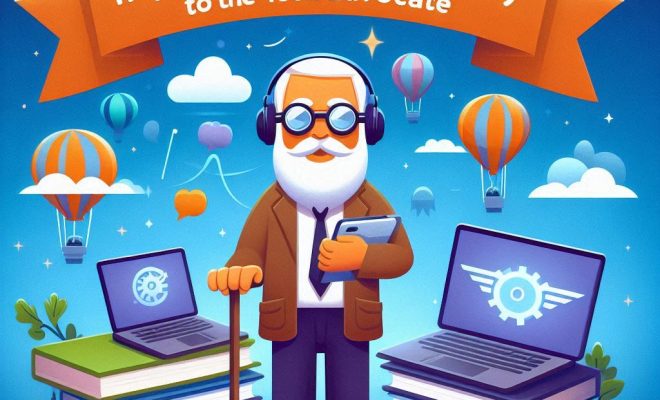
Introduction:
A calculator is an invaluable tool when it comes to solving mathematical problems quickly and accurately. While there are many types of calculators available in the market, it can be an interesting and rewarding experience to create one yourself. In this article, we will guide you through the process of making a simple calculator using basic programming skills.
Step 1: Choose a Programming Language
The first step in creating a calculator is to select an appropriate programming language. Some popular choices include Python, JavaScript, and Java. If you’re new to programming or unsure which language to choose, consider starting with Python due to its simplicity and readability.
Step 2: Set Up Your Development Environment
Once you’ve chosen a programming language, you’ll need to set up a development environment where you can write and test your code. Depending on your programming language choice, there are various Integrated Development Environments (IDEs) available. For example, you can use PyCharm for Python or Visual Studio Code for JavaScript.
Step 3: Understand Basic Mathematical Operations
Before diving into coding, familiarize yourself with the four basic mathematical operations: addition, subtraction, multiplication, and division. These operations will form the core functionality of your calculator.
Step 4: Write the Core Functions
Start by writing functions for each of the basic mathematical operations. Here’s an example using Python:
“`python
def add(a, b):
return a + b
def subtract(a, b):
return a – b
def multiply(a, b):
return a * b
def divide(a, b):
if b == 0:
return “Error: Cannot divide by zero”
else:
return a / b
“`
Step 5: Create a User Interface (Optional)
While not strictly necessary for a functioning calculator, adding a user interface can greatly improve user experience. For a basic calculator, consider creating a command-line interface. Here’s an example using Python:
“`python
def main():
operation = input(“Enter operation (+, -, *, /): “)
num1 = float(input(“Enter first number: “))
num2 = float(input(“Enter second number: “))
if operation == “+”:
print(add(num1, num2))
elif operation == “-“:
print(subtract(num1, num2))
elif operation == “*”:
print(multiply(num1, num2))
elif operation == “/”:
print(divide(num1, num2))
else:
print(“Invalid operation. Please try again.”)
if __name__ == “__main__”:
main()
“`
Step 6: Test Your Calculator
Before considering your calculator complete, thoroughly test it to ensure all functions and features work correctly. Be sure to test various combinations of numbers and operations to identify any bugs or errors in your code.
Conclusion:
By following this step-by-step guide, you should now have a basic understanding of how to create a calculator using programming skills. While this is just the beginning, feel free to expand on this project by adding new functionalities like memory functions, trigonometric functions, or even creating a graphical user interface (GUI). The possibilities are endless!