How to Install PHPMailer
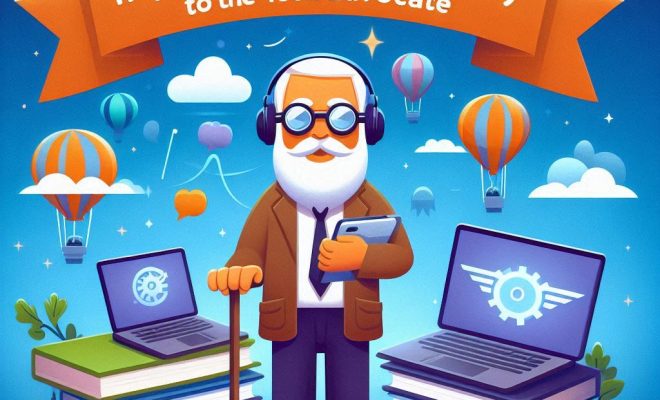
PHPMailer is a powerful, popular, and easy-to-use library that allows you to send emails from your PHP applications. In this article, we’ll guide you through the process of installing PHPMailer so that you can start sending emails with ease.
Step 1: Download PHPMailer
To begin, download the latest version of PHPMailer from the official GitHub repository at https://github.com/PHPMailer/PHPMailer. Click on the green “Code” button and then choose “Download ZIP.” Save the file to your computer and extract the contents in a folder of your choice.
Step 2: Upload PHPMailer files to your server
Next, upload the extracted PHPMailer files to your web server using an FTP client or any other method that works for you. You may place them in a specific folder or directly in your project directory, but be sure to remember the location for future reference.
Step 3: Include PHPMailer in your project
In your PHP script, you need to include the PHPMailer library before using it. To do this, use the `require` or `require_once` function as shown below:
“`php
require ‘path/to/PHPMailer/src/PHPMailer.php’;
require ‘path/to/PHPMailer/src/SMTP.php’;
“`
Remember to replace ‘path/to’ with the actual path where you uploaded the PHPMailer files in Step 2.
Step 4: Create a new instance of PHPMailer
To use PHPMailer for sending emails, create a new instance of the `PHPMailer` class. This can be done by adding the following code in your PHP script:
“`php
$mail = new PHPMailer\PHPMailer\PHPMailer();
“`
Step 5: Configure email settings
Now it’s time to configure the email settings. Depending on your requirements and email service provider, these settings may vary. However, the following code shows a basic example for setting up email sending with SMTP:
“`php
$mail->isSMTP();
$mail->Host = ‘smtp.example.com’; // Replace with your SMTP server
$mail->SMTPAuth = true;
$mail->Username = ‘[email protected]’; // Replace with your email address
$mail->Password = ‘your-password’; // Replace with your email password
$mail->SMTPSecure = ‘tls’;
$mail->Port = 587;
$mail->setFrom(‘[email protected]’, ‘Mailer’); // Replace with your sender email and name
$mail->addAddress(‘[email protected]’, ‘John Doe’); // Replace with recipient’s email and name
“`
Step 6: Prepare and send the email
Lastly, set the email subject, body, and any other necessary information. Then, use the `send` method to send the email. Here’s an example:
“`php
$mail->Subject = ‘Hello World!’;
$mail->Body = ‘This is a test email sent using PHPMailer.’;
if ($mail->send()) {
echo ‘Message has been sent’;
} else {
echo “Message could not be sent. Mailer Error: {$mail->ErrorInfo}”;
}
“`
And that’s it! You have successfully installed and used PHPMailer to send an email from your PHP application. For more advanced features and options, refer to the PHPMailer documentation at https://github.com/PHPMailer/PHPMailer/wiki.