How to Flatten a Nested List in Python
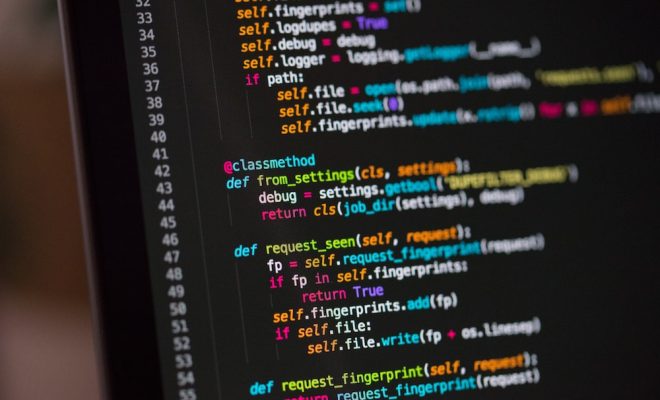
Python is a popular programming language that can be used to manipulate data in various ways. Nested lists are a common data structure in Python, and they can be used to represent complex data structures. However, at times it may be required to flatten a nested list in Python to perform further processing. In this tutorial, we will explain how to flatten a nested list in Python.
What is a Nested List in Python?
A list in Python is a collection of elements that are ordered and mutable. A nested list is a list that contains other lists as its elements. Nested lists can be used to represent complex data structures such as a matrix, a tree, or a graph.
Here’s an example of a nested list in Python:
“`python
nested_lst = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
“`
This is a list that contains three other lists as its elements. Each of these lists contains three integers.
How to Flatten a Nested List in Python?
Flattening a nested list means flattening all the nested elements in the list so that we have a single list containing all the elements of the nested lists. Here’s how to do it in Python:
Method 1: Using List Comprehension
One way to flatten a nested list is by using list comprehension. List comprehension is a concise way to create lists in Python. Here’s how to use list comprehension to flatten a nested list:
“`python
nested_lst = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# Using list comprehension to flatten the nested list
flat_lst = [elem for lst in nested_lst for elem in lst]
print(flat_lst)
“`
Output:
“`
[1, 2, 3, 4, 5, 6, 7, 8, 9]
“`
Explanation:
In the above code, we have used list comprehension to iterate over each element in each list inside the nested list. Then we have added the element to a new list called flat_lst. Finally, we have printed the flat_lst.
Method 2: Using Nested for Loops
Another way to flatten a nested list is by using nested for loops. Here’s how to use nested for loops to flatten a nested list:
“`python
nested_lst = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# Using nested for loops to flatten the nested list
flat_lst = []
for l in nested_lst:
for elem in l:
flat_lst.append(elem)
print(flat_lst)
“`
Output:
“`
[1, 2, 3, 4, 5, 6, 7, 8, 9]
“`
Explanation:
In the above code, we have used nested for loops to iterate over each element in each list inside the nested list. Then we have added the element to a new list called flat_lst. Finally, we have printed the flat_lst.
Conclusion
Flattening a nested list in Python can be done using list comprehension or nested for loops. Both methods are efficient and can be used depending on the programmer’s preference. By using either method, you can now flatten a nested list in Python with ease.