How to Create a Simple Image Gallery Using HTML, CSS, and JavaScript
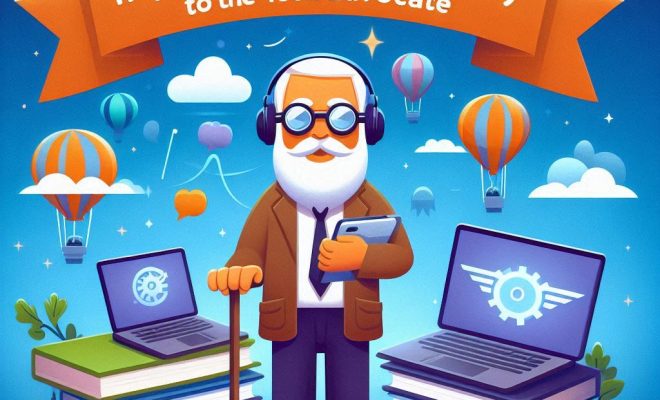
Creating a simple image gallery can be a great way to showcase your work or highlight special photos on your website. Luckily, creating an image gallery is easier than you might think. With just a few lines of code using HTML, CSS, and JavaScript, you can create a simple yet effective image gallery that will impress your visitors. Here’s how to do it.
Step 1: Create Your HTML Structure
The first step in creating your image gallery is to create the HTML structure. This will determine how your images will be displayed on your website. Start by opening a new HTML file in your text editor and adding the following code:
“`html
“`
This HTML code creates a basic structure for your website and includes five images that you will use in your gallery. Be sure to replace the image filenames with your own images. Save this file as index.html.
Step 2: Style Your Gallery
Next, you will need to style your gallery using CSS. Open a new file in your text editor and add the following code:
“`css
.gallery {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(200px, 1fr));
grid-gap: 20px;
}
.gallery img {
width: 100%;
height: auto;
border-radius: 5px;
}
.gallery img:hover {
opacity: 0.8;
}
“`
This CSS code creates a grid of images that will be displayed on your website. The `display: grid;` property creates a grid layout, while `grid-template-columns: repeat(auto-fit, minmax(200px, 1fr));` sets the minimum and maximum width for each image. The `grid-gap: 20px;` adds spacing between the images. The `gallery img` CSS block styles each image, setting the width to 100% and adding a border radius. Finally, the `gallery img:hover` block makes each image slightly transparent when the mouse hovers over it. Save this file as style.css.
Step 3: Add JavaScript Functionality
Your image gallery is almost complete! You just need to add a little bit of JavaScript to make it work. Open a new file in your text editor and add the following code:
“`javascript
const gallery = document.querySelector(“.gallery”);
const images = gallery.querySelectorAll(“img”);
let current = 0;
function showImage(index) {
if (index >= images.length) {
current = 0;
} else if (index < 0) {
current = images.length – 1;
} else {
current = index;
}
images.forEach(image => image.classList.remove(“active”));
images[current].classList.add(“active”);
}
showImage(current);
setInterval(() => {
showImage(current + 1);
}, 5000);
“`
This JavaScript code creates an image gallery slideshow that changes every 5 seconds. The `gallery` variable selects the gallery container, and the `images` variable selects all of the images within the gallery container. The `current` variable keeps track of the current image being displayed. The `showImage` function displays the next image in the slideshow by adding and removing the `active` class on each image. Finally, the `setInterval` function runs the `showImage` function every 5 seconds.
Step 4: Test Your Image Gallery
Congratulations, your image gallery is complete! All that’s left is to test it out. Save your script.js file and open the index.html file in your web browser. You should see a grid of images with a slideshow that automatically plays in the background. Use your mouse to hover over the images and see the opacity change.
In conclusion, creating a simple image gallery using HTML, CSS, and JavaScript can be easy and fun. With just a little bit of code, you can showcase your work or highlight special photos on your website. Follow these four steps, and you’ll be on your way to creating an impressive image gallery in no time!