How to Code a Physics-Based Character Controller in Unity3D
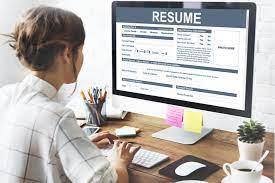
Unity3D is a popular game engine that has a wide range of features that can be used to create stunning games. Physics-based character controllers are one such feature. They allow the player’s movements to be affected by physics forces such as gravity, so the character moves more realistically. This article will provide a step-by-step guide on how to code a physics-based character controller in Unity3D.
Step 1: Create a Unity Project
The first step is to create a new Unity project. To create a new Unity project, open the Unity Hub and click the “New” button. You will be prompted to enter a project name, location, and other details. Keep in mind that the character controller will be a part of this project.
Step 2: Add a Rigidbody Component
The Rigidbody component is an important component when creating a physics-based character controller. It allows the character to be affected by forces such as gravity, which is essential for creating realistic movements. To add a Rigidbody component, select the character object in the hierarchy, and click the “Add Component” button in the Inspector. From the drop-down menu, select “Physics > Rigidbody.”
Step 3: Add a Capsule Collider Component
The Capsule Collider component is another important component that is needed to create a physics-based character controller. It defines the character’s shape and size, which is important for collision detection. To add a Capsule Collider component, select the character object in the hierarchy, and click the “Add Component” button in the Inspector. From the drop-down menu, select “Physics > Capsule Collider.”
Step 4: Write the Character Controller Script
Now it’s time to write the script that will control the character. The script will need to access the Rigidbody component and use it to apply forces to the character. It will also need to read input from the player and use that input to move the character. In the script, you will need to declare variables for the Rigidbody component and for the speed of the character.
Here is an example script:
public class CharacterController : MonoBehaviour {
public float speed;
private Rigidbody rb;
// Start is called before the first frame update
void Start() {
rb = GetComponent();
}
// Update is called once per frame
void Update() {
float moveHorizontal = Input.GetAxis(“Horizontal”);
float moveVertical = Input.GetAxis(“Vertical”);
Vector3 movement = new Vector3(moveHorizontal, 0.0f, moveVertical);
rb.AddForce(movement * speed);
}
}
In this script, the Update() function is called once per frame and reads input from the player using Input.GetAxis(). This function returns a value between -1 and 1, which represents the direction and magnitude of the input. The movement vector is created using this input and is scaled by the speed variable. Then, the AddForce() function is used to apply a force to the Rigidbody component, which will move the character.
Step 5: Drag the Script to the Character Object
Now that the script is written, it needs to be attached to the character object. To do this, drag the script from the project window onto the character object in the hierarchy. This will attach the script to the character object.
Step 6: Play the Game and Test the Character Controller
Now that everything is set up, it’s time to test the character controller. To do this, press the “Play” button in the Unity editor. Move the character using the arrow keys or WASD keys and observe how the character moves.
Conclusion
This article has provided a step-by-step guide on how to code a physics-based character controller in Unity3D. The process involves adding a Rigidbody component, a Capsule Collider component, and writing a script to control the character. With these steps completed, you should now be able to create a functioning character controller that uses physics forces for more realistic movement.