How to calculate mean in r
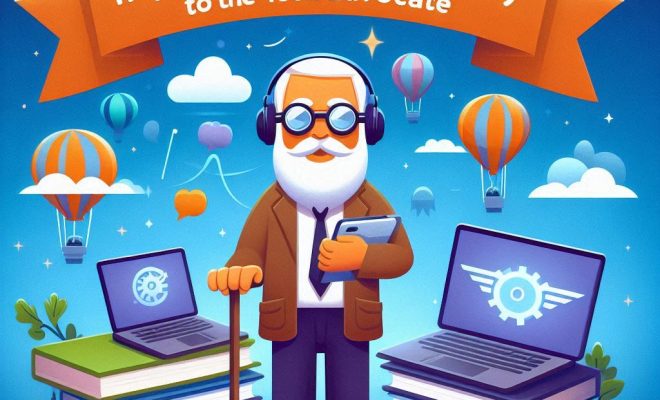
Introduction
Mean, also known as average, is a fundamental concept in statistics and a widely used measure of central tendency. It is simple to understand and easy to calculate. In this article, we will explore how to calculate mean in R, a popular programming language for data analysis.
What is Mean?
Mean is the sum of all observations divided by the total number of observations. In layman’s terms, it represents the “average” value of a dataset. It can be expressed using the following formula:
Mean = (Sum of all observations) / (Number of observations)
Calculating Mean in R
R provides several built-in functions and libraries that make it easy to calculate the mean of a dataset. The most common method to calculate the mean in R is using the mean() function on a numeric vector or data frame.
1. Using mean() function on numeric vectors:
To calculate the mean of a numeric vector, simply pass it as an argument to the mean() function, like this:
“`R
numbers <- c(1, 2, 3, 4, 5)
mean_value <- mean(numbers)
print(mean_value) # Output: 3
“`
2. Using mean() function on data frames:
To compute the mean for specific columns in a data frame, you can use the colMeans() or sapply() functions along with the mean() function. Here’s how:
a) colMeans() function:
“`R
data_frame <- data.frame(column1 = c(1, 2, 3), column2 = c(4, 5, 6))
mean_values <- colMeans(data_frame)
print(mean_values)
“`
b) sapply() function:
“`R
data_frame <- data.frame(column1 = c(1, 2, 3), column2 = c(4, 5, 6))
mean_values <- sapply(data_frame, mean)
print(mean_values)
“`
Handling Missing Values (NA) in R
Sometimes datasets contain missing values, which are represented as NA in R. By default, the mean() function returns NA if any value in the dataset is NA. To handle these missing values and calculate the mean of available data, use the `na.rm` argument and set it to TRUE:
“`R
numbers_with_missing_values <- c(1, 2, 3, NA, 4)
mean_value <- mean(numbers_with_missing_values, na.rm = TRUE)
print(mean_value) # Output: 2.5
“`
Conclusion
Calculating the mean in R is quick and straightforward using the mean() function. The function can be easily applied to numeric vectors and data frames. It also offers a convenient way to handle missing values through the `na.rm` argument.
Understanding and calculating the mean is crucial for anyone working with data in R. Now that you know how to calculate the mean in R go ahead and explore more advanced statistics functions like median, mode, and standard deviation to enhance your data analysis skills.