C++ String Methods You Should Master Today
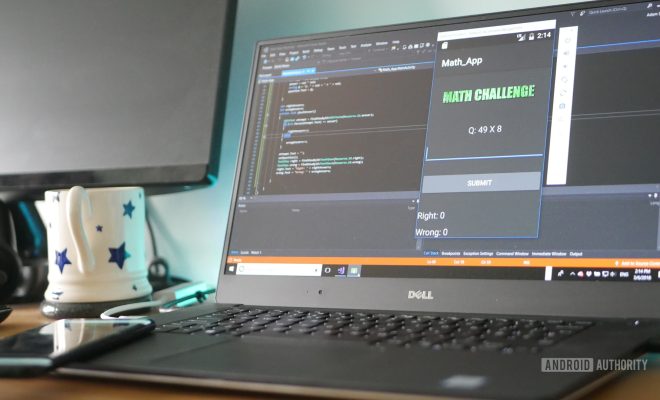
C++ is a powerful and versatile programming language that is widely used for developing applications in a variety of sectors. When working with C++, mastering string methods is essential for producing reliable and efficient code. Here are some of the C++ string methods that you should master today.
1. append()
The append() method is used to add a string or a character to the end of an existing string. It is a fast and efficient way of concatenating two strings. The syntax for using the append() method is as follows:
string str1 = “Hello “;
string str2 = “World!”;
str1.append(str2);
The result of the above code would be a new string “Hello World!”.
2. substr()
The substr() method is used to extract a portion of a string. It takes two parameters, the start index and the length of the substring. The syntax for using the substr() method is as follows:
string str = “Hello World!”;
string substr = str.substr(0, 5);
The result of the above code would be a new string “Hello”.
3. size()
The size() method returns the length of the string. It is a simple and efficient way of determining the size of a string. The syntax for using the size() method is as follows:
string str = “Hello World!”;
int len = str.size();
The result of the above code would be the integer value 12, which is the length of the string “Hello World!”.
4. find()
The find() method is used to search for a substring within a string. It takes a single parameter, which is the substring to search for. The method returns the index of the first occurrence of the substring within the string. If the substring is not found, the method returns the value -1. The syntax for using the find() method is as follows:
string str = “Hello World!”;
int index = str.find(“World”);
The result of the above code would be the integer value 6, which is the index of the substring “World” within the string “Hello World!”.
5. replace()
The replace() method is used to replace a portion of a string with a new string or character. It takes three parameters, the start index, the length of the substring to replace, and the replacement string. The syntax for using the replace() method is as follows:
string str = “Hello World!”;
str.replace(6, 5, “Universe”);
The result of the above code would be a new string “Hello Universe!”.
In conclusion, mastering these C++ string methods will help you to develop efficient and reliable applications. These methods are widely used in C++ programming, and their versatility makes them essential for developing applications in a variety of sectors.