Build a Music Search Application With React and Spotify API
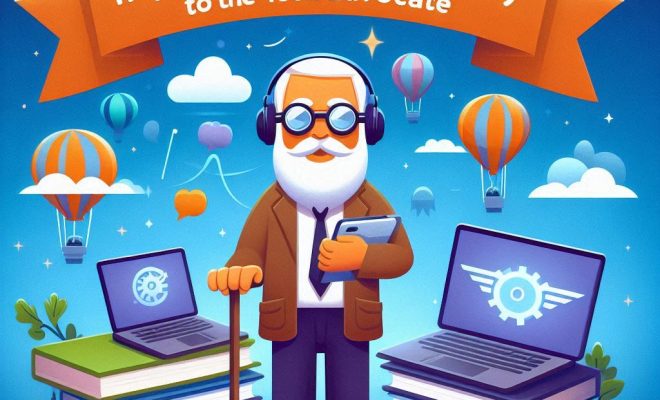
As music lovers, we often find ourselves searching for a new song or artist to add to our playlist. With thousands of songs available through Spotify, it can be challenging to sift through all the options.
But what if we had a music search application that made finding new music a breeze? In this article, we’ll explore how to build a music search application using React and the Spotify API.
Getting Started
Before we dive into the code, let’s familiarize ourselves with the Spotify API. The API allows us to access information about artists, albums, tracks, and playlists. We can use this data to build a music search application that returns relevant results to users.
To get started, we need to create a Spotify developer account and obtain an API key. The steps are straightforward and explained in detail on the Spotify Developer website.
Once we have our API key, we can begin building our application using React.
Building the Music Search Application
First, we need to set up our project’s file structure and dependencies. We’ll use Create React App, which comes with a predefined file structure and preconfigured settings for building React applications.
We’ll also need the Spotify Web API package, which we can install using the following command:
“`
npm install spotify-web-api-js
“`
Next, let’s define our component’s state and add our search bar input field.
“`
class SearchBar extends React.Component {
constructor(props) {
super(props);
this.state = {
query: “”,
};
this.handleSubmit = this.handleSubmit.bind(this);
this.handleChange = this.handleChange.bind(this);
}
handleChange(event) {
this.setState({ query: event.target.value });
}
handleSubmit(event) {
event.preventDefault();
this.props.onSearch(this.state.query);
}
render() {
return (
<input
type=”text”
placeholder=”Search for an artist, song, or album”
value={this.state.query}
onChange={this.handleChange}
/>
);
}
}
“`
Our `SearchBar` component has a `query` state that stores the user’s search query. We’ve added an input field for users to enter their search query and a submit event that passes the query to the parent component.
Let’s now create a `Spotify` component that connects to the Spotify API.
“`
const Spotify = new SpotifyWebApi();
class App extends React.Component {
constructor(props) {
super(props);
this.state = {
tracks: [],
albums: [],
artists: [],
};
this.search = this.search.bind(this);
}
componentDidMount() {
Spotify.setAccessToken(YOUR_ACCESS_TOKEN);
}
search(query) {
Spotify.search(query, [“track”, “album”, “artist”]).then(
(response) => {
this.setState({
tracks: response.tracks.items,
albums: response.albums.items,
artists: response.artists.items,
});
}
);
}
render() {
return (
);
}
}
“`
Our `Spotify` component creates a new instance of the `SpotifyWebApi` class and sets our access token. We’ve also defined a `search` function that passes the user’s search query to the Spotify API and returns the results.
Finally, we’ve added `TrackList`, `AlbumList`, and `ArtistList` components that render the results of our search query.
Conclusion
In just a few lines of code, we’ve built a functional music search application that uses the Spotify API to return relevant results. We can now add additional features, such as the ability to play tracks or view album artwork. With React and the Spotify API, the possibilities for building music applications are endless. Happy coding! </input