How I write code using Cursor
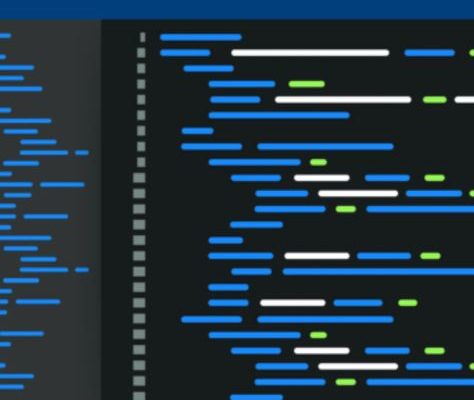
Cursors are crucial in many programming languages and database management systems, especially when manipulating data. They allow developers to traverse and manipulate records one at a time. Here’s how to effectively use cursors in your code
Understanding Cursors
A cursor acts as a pointer to the result set of a database query. It enables row-by-row processing of the result set, allowing you to perform operations on each record. This is particularly useful for complex data manipulations or when detailed processing is required.
Declaring a Cursor
To write code using a cursor, you start by declaring it. The declaration defines the SQL statement that the cursor will execute to retrieve the desired records.
“`sql
DECLARE cursor_name CURSOR FOR
SELECT column1, column2 FROM table_name WHERE condition;
“`
Opening the Cursor
Once declared, the next step is to open the cursor. This command executes the SQL statement and makes the result set available for processing.
“`sql
OPEN cursor_name;
“`
Fetching Data
After opening a cursor, you can retrieve each row using the FETCH command. Typically, you will fetch data into predefined variables.
“`sql
FETCH NEXT FROM cursor_name INTO @variable1, @variable2;
“`
Processing Data
You can encapsulate the fetch operation within a loop to process each row. This loop continues until all records are processed.
“`sql
WHILE @@FETCH_STATUS = 0
BEGIN
— Process the data here
— Fetch the next record
FETCH NEXT FROM cursor_name INTO @variable1, @variable2;
END;
“`
Closing and Deallocating
After processing the records, it is essential to close and deallocate the cursor to free up resources.
“`sql
CLOSE cursor_name;
DEALLOCATE cursor_name;
“`
Conclusion
Cursors are powerful tools in database programming. By following the steps outlined above, you can efficiently write code that manipulates data iteratively, enhancing your application’s functionality and performance. Remember, while cursors are useful, they can impact performance, so always consider set-based operations first when possible.