How to Execute HTTP POST Requests in Android
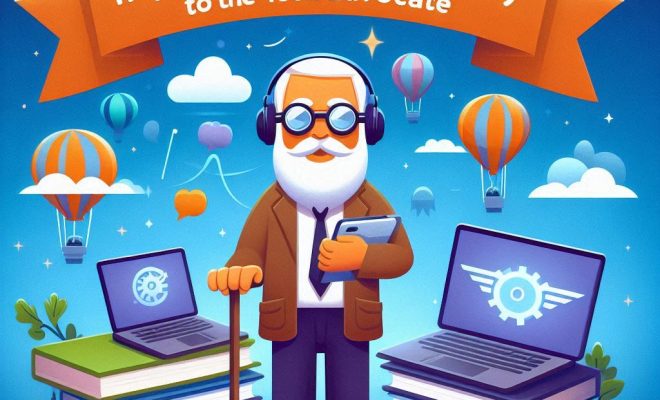
Introduction
HTTP POST is a common method used when working with APIs and web services to send data from an Android app to a server. In this article, we will discuss how to execute HTTP POST requests in Android using different libraries and techniques.
1. HttpURLConnection
The most straightforward way to execute an HTTP POST request in Android is by using HttpURLConnection, which is available since API level 1.
Steps:
1. Create a new URL object with the target endpoint.
2. Open a connection using the URL object.
3. Set the request method as “POST”.
4. Add any necessary headers (Content-Type, Authorization, etc.).
5. Write the data to send in the request body.
6. Read and process the server response.
Example:
URL url = new URL(“https://api.example.com/posts”);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod(“POST”);
connection.setRequestProperty(“Content-Type”, “application/json; utf-8”);
connection.setRequestProperty(“Accept”, “application/json”);
// Send data
String jsonInputString = “{\”title\”:\”My Post\”,\”content\”:\”Hello World!\”}”;
try (OutputStream os = connection.getOutputStream()) {
byte[] input = jsonInputString.getBytes(“utf-8”);
os.write(input, 0, input.length);
}
// Process response
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader br = new BufferedReader(new InputStreamReader(connection.getInputStream(), “utf-8”));
StringBuilder responseBuilder = new StringBuilder();
String responseLine;
while ((responseLine = br.readLine()) != null) {
responseBuilder.append(responseLine.trim());
}
String responseBody = responseBuilder.toString();
}
2. OkHttpClient Library
An alternative to HttpURLConnection is OkHttpClient provided by Square OkHttp Library, which helps to simplify HTTP requests as well as optimizes performance.
Steps:
1. Add OkHttp to your project dependencies.
2. Create an OkHttpClient instance.
3. Create a RequestBody with the desired content type and data.
4. Build an HTTP POST Request using the Request.Builder class.
5. Execute the request and process the response.
Example:
In your build.gradle file, add:
dependencies {
implementation ‘com.squareup.okhttp3:okhttp:4.9.2’
}
In your Java code:
“`java
OkHttpClient client = new OkHttpClient();
JSONObject json = new JSONObject();
json.put(“title”, “My Post”);
json.put(“content”, “Hello World!”);
RequestBody body = RequestBody.create(json.toString(), MediaType.parse(“application/json; charset=utf-8”));
Request request = new Request.Builder()
.url(“https://api.example.com/posts”)
.post(body)
.build();
client.newCall(request).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
// Handle failure
}
@Override
public void onResponse(Call call, Response response) throws IOException {
// Process server response
String responseBody = response.body().string();
}
});
Conclusion
Executing HTTP POST requests is essential for interacting with APIs and web services in Android applications. HttpURLConnection and OkHttpClient are two popular choices among developers, with OkHttpClient being a more modern alternative offering better performance. Choose the right approach based on your specific needs to create efficient and robust Android apps.